如何在 C# 中读取 JSON 数据(使用控制台应用程序和 ASP.NET MVC 的示例)?
如今,JSON 由于其简单性和轻量级而被广泛用于交换数据,因此在本文中,我将为您提供一个示例,其中包含在 C# (.NET/.NET Core) 中读取和解析 JSON 数据的代码,我将使用多个示例,ASP.NET MVC 和控制台应用程序、.NET Core 和动态 JSON 解析示例。
您可以将 C# 代码用于任何应用程序,无论是 C# 控制台应用程序、Windows 应用程序还是 ASP.NET Web 应用程序。
MVC中C#读取JSON数据
在本文中,我将执行以下步骤:
获取示例 JSON
创建 JSON 的类文件。
使用 DeserializeObject 反序列化 JSON 并将其保存为步骤 2 创建的类列表。
使用模型在视图中打印 JSON。
第 1 步:在继续执行任何步骤之前,您需要在 Visual Studio 中创建一个 ASP.NET MVC 示例项目,因此导航到“文件”->“新建”->“项目”-> 从左侧窗格中选择“Web”和“ASP” .NET Web 应用程序”(右窗格),为其命名并单击“确定”
单击“确定”后,将出现新窗口,让我们从中选择“MVC”模板,为我们的项目自动生成基本的 MVC 配置。
第 2 步:获取示例 JSON,这是我将使用的示例 JSON
{
"name": "John Smith",
"sku": "20223",
"price": 23.95,
"shipTo": {
"name": "Jane Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": "12345"
},
"billTo": {
"name": "John Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": "12345"
}
}
注意:您始终可以使用 JSONLint 等网站验证您的 JSON
考虑到上面的 JSON,我们可以创建一个 C# 类来在其中保存数据,然后将其用作模型并在视图中打印。
为此,您可以使用 Visual-studio 的“粘贴为特殊”功能,该功能对于转换很有用,因此使用帖子“将 JSON 转换为类对象的快速提示”中提供的示例,在模型中创建一个类“JSONModel.cs”项目文件夹,然后复制上面的示例 JSON,导航到 “编辑”->“选择性粘贴”->“将 JSON 粘贴为类” ,如下图所示。
那么你的 C# 类将如下所示
namespace ReadJSONusingCsharp.Models
{
public class JSONModel
{
public class Rootobject
{
public string name { get; set; }
public string sku { get; set; }
public float price { get; set; }
public Shipto shipTo { get; set; }
public Billto billTo { get; set; }
}
public class Shipto
{
public string name { get; set; }
public string address { get; set; }
public string city { get; set; }
public string state { get; set; }
public string zip { get; set; }
}
public class Billto
{
public string name { get; set; }
public string address { get; set; }
public string city { get; set; }
public string state { get; set; }
public string zip { get; set; }
}
}
}
现在,转到项目的 HomeController 和 Index ActionMethod 内,使用下面的 C# 代码来反序列化 JSON 并将其转换为类模型。
using Newtonsoft.Json;
using ReadJSONusingCsharp.Models;
using System.Web.Mvc;
namespace ReadJSONusingCsharp.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
//get JSOn string
var json = "{ \"name\": \"John Smith\", \"sku\": \"20223\",\"price\": 23.95," +
"\"shipTo\": { \"name\": \"Jane Smith\", \"address\": \"123 Maple Street\", \"city\": \"Pretendville\",\"state\": \"NY\", \"zip\": \"12345\" }, " +
"\"billTo\": {\"name\": \"John Smith\",\"address\": \"123 Maple Street\",\"city\": \"Pretendville\",\"state\": \"NY\",\"zip\": \"12345\" }}";
//Convert JSON into Model
JSONModel.Rootobject records = JsonConvert.DeserializeObject<JSONModel.Rootobject>(json); // JSON.Net
//return data as Model to view
return View(records);
}
}
}
在 Index.cshtml 视图中使用 Model 来获取数据并将其打印为 HTML。
@model ReadJSONusingCsharp.Models.JSONModel.Rootobject
@{
ViewBag.Title = "Home Page";
}
<p>
After reading JSON, it's output is as below
</p>
<p>Name : @Model.name</p>
<p>SKU: @Model.sku</p>
<p>Price : @Model.price</p>
<hr />
<p>Ship to name : @Model.shipTo.name</p>
<p>Ship to address: @Model.shipTo.address</p>
<p>Ship to City: @Model.shipTo.city</p>
<p>Ship to State: @Model.shipTo.state</p>
<p>Ship to Zip: @Model.shipTo.zip</p>
<hr />
<p>Billed to name : @Model.billTo.name</p>
<p>Billed to address: @Model.billTo.address</p>
<p>Billed to City: @Model.billTo.city</p>
<p>Billed to State: @Model.billTo.state</p>
<p>Billed to Zip: @Model.billTo.zip</p>
在浏览器中构建并运行它,您将得到如下输出
使用控制台应用程序读取 C# 中的 JSON 数据。
我们将遵循与 MVC 项目中使用的相同过程,通过导航到文件 -> 新建 -> 项目 -> 选择“Window Classic 桌面”(左窗格)和“控制台应用程序”(从右开始)来创建一个新的控制台应用程序项目窗格),提供名称(“ReadJSONInCharp”),然后单击“确定”
我们必须在 MVC 项目中创建与 JSONModel.cs 相同的类,因此右键单击解决方案中的项目,选择“添加”-> 选择“类”,将其命名为“JSONModel.cs”并使用下面的 C# 代码
namespace ReadJSONInCharp
{
public class JSONModel
{
public class Rootobject
{
public string name { get; set; }
public string sku { get; set; }
public float price { get; set; }
public Shipto shipTo { get; set; }
public Billto billTo { get; set; }
}
public class Shipto
{
public string name { get; set; }
public string address { get; set; }
public string city { get; set; }
public string state { get; set; }
public string zip { get; set; }
}
public class Billto
{
public string name { get; set; }
public string address { get; set; }
public string city { get; set; }
public string state { get; set; }
public string zip { get; set; }
}
}
}
其中一个额外步骤是使用 Nuget 包管理器在项目中安装 NewtonSoft.JSON,导航到“工具”->“Nuget 包管理器”-> 选择“管理解决方案的 nuget 包...”,然后单击“浏览” ”选项卡,搜索“NewtonSoft.JSON”并选择它并安装在您的项目中
转到主Program.cs文件并使用下面的代码
using Newtonsoft.Json;
using System;
namespace ReadJSONInCharp
{
class Program
{
static void Main(string[] args)
{
var json = "{ \"name\": \"John Smith\", \"sku\": \"20223\",\"price\": 23.95," +
"\"shipTo\": { \"name\": \"Jane Smith\", \"address\": \"123 Maple Street\", \"city\": \"Pretendville\",\"state\": \"NY\", \"zip\": \"12345\" }, " +
"\"billTo\": {\"name\": \"John Smith\",\"address\": \"123 Maple Street\",\"city\": \"Pretendville\",\"state\": \"NY\",\"zip\": \"12345\" }}";
JSONModel.Rootobject record = JsonConvert.DeserializeObject<JSONModel.Rootobject>(json); // JSON.Net
Console.WriteLine("JSON details");
Console.WriteLine(record.name);
Console.WriteLine(record.price);
Console.WriteLine(record.sku);
Console.WriteLine();
Console.WriteLine("Ship to details");
Console.WriteLine(record.shipTo.name);
Console.WriteLine(record.shipTo.address);
Console.WriteLine(record.shipTo.city);
Console.WriteLine(record.shipTo.state);
Console.WriteLine(record.shipTo.zip);
Console.WriteLine();
Console.WriteLine("Bill to details");
Console.WriteLine(record.billTo.name);
Console.WriteLine(record.billTo.address);
Console.WriteLine(record.billTo.city);
Console.WriteLine(record.billTo.state);
Console.WriteLine(record.billTo.zip);
Console.ReadLine();
}
}
}
构建并运行您的应用程序,您将得到如下输出
我们就这样完成了,但是有时您的 JSON 对象可能是动态的,所以让我们看另一个示例。
在 C# 中读取动态 JSON 数据
现在,假设您的 JSON 是动态的,因此您无法创建它的类,那么如何在没有模型的情况下访问它呢?
我将使用这个问题中提供的答案“ How to conversiondynamic JSON string into C# class? ”,这对我来说很有用,所以从答案来看,如果你的 JSON 如下
{
"Items": [{
"Name": "Apple",
"Price": 12.3
},
{
"Name": "Grape",
"Price": 3.21
}
],
"Date": "21/11/2010"
}
您可以将其反序列化为动态对象,然后像访问数组一样访问数据。
为此,您需要在应用程序中安装NewtonSoft.JSON来自 NuGet 的软件包,然后您可以使用下面的 C# 代码。
string jsonString = "{\"Items\": [{\"Name\": \"Apple\",\"Price\": 12.3},{\"Name\": \"Grape\",\"Price\": 3.21}],\"Date\": \"21/11/2010\"}";
dynamic DynamicData = JsonConvert.DeserializeObject(jsonString);
Console.WriteLine( DynamicData.Date); // "21/11/2010"
Console.WriteLine(DynamicData.Items.Count); // 2
Console.WriteLine(DynamicData.Items[0].Name); // "Apple"
Console.WriteLine(DynamicData.Items[0].Price);
Console.WriteLine(DynamicData.Items[1].Name);
Console.WriteLine(DynamicData.Items[1].Price);// 3.21 (as a decimal)
输出:
上面问题的答案中提供了其他几种方法,另一种如下
dynamic details= JObject.Parse("{ 'Name': 'Jaya', 'Address': { 'City': 'New Delhi', 'State': 'Delhi' }, 'Age': 31}");
string name = details.Name; //GET Name
string address = details.Address.City; //Get City
我们已经完成了示例,如果您有任何疑问,请随时在下面评论。
在 .NET 6 中读取或解析 JSON
在 .NET 6 中,我们可以使用System.Text.Json命名空间,以与 Newtonsoft.Json 类似的方式使用新类 JsonObject、JsonArray、JsonValue 和 JsonNode 来启用 DOM 解析、导航和操作。
以下是在 C# 中使用 .NET 6 解析 JSON 的完整示例
using System.Text.Json;
string data = "{\"Items\": [{\"Name\": \"Apple\",\"Price\": 12.3},{\"Name\": \"Grape\",\"Price\": 3.21}],\"Date\": \"21/11/2010\"}";
JsonDocument doc = JsonDocument.Parse(data);
JsonElement root = doc.RootElement;
var names = new List<string>();
Enumerate(root);
void Enumerate(JsonElement element)
{
if (element.ValueKind == JsonValueKind.Object)
{
foreach (var item in element.EnumerateObject())
{
if (item.Value.ValueKind == JsonValueKind.Object)
{
Enumerate(item.Value);
}
else if(item.Value.ValueKind == JsonValueKind.Array)
{
var root = item.Value;
var u1 = root[0];
var u2 = root[1];
Console.WriteLine(u1);
Console.WriteLine(u2);
Console.WriteLine(u1.GetProperty("Name")); // Apple
Console.WriteLine(u1.GetProperty("Price")); //12.3
Console.WriteLine(u2.GetProperty("Name")); //Grape
Console.WriteLine(u2.GetProperty("Price")); //3.21
}
else
{
Console.WriteLine(item.Value.GetRawText()); // 21/11/2010
}
}
}
}
这是输出
注意:与 MVC/WebAPI 请求和模型绑定器不同,当使用您自己的代码时,System.Text.Json 不会自动处理驼峰式 JSON 属性,因此您需要为其提供代码
JsonSerializerOptions options = new JsonSerializerOptions
{
PropertyNamingPolicy = JsonNamingPolicy.CamelCase, // set camelCase
WriteIndented = true // write pretty json
};
// pass options to serializer
var json = JsonSerializer.Serialize(jsonDataToSerialize, options);
// pass options to deserializer
var order = JsonSerializer.Deserialize<ModelClass>(json, options);
System.Text.Json还可以作为 NuGet 包用于 .Net Framework 和 .Net Standard。
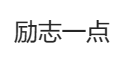