在 C# 中将列表转换为字典(多种方式)
使用 Linq
在 C# 中将列表转换为字典的简单快捷方法之一是使用 Lambda
using System;
using System.Linq;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
//create list of students type
List<Students> studentList = new List<Students> {
new Students(){Id = 208, Name = "John"},
new Students(){Id = 209, Name = "Wick"},
new Students(){Id = 210, Name = "Morbius"},
new Students(){Id = 211, Name = "Geralt"}
};
//convert list into dictionary
// key as Id
//value as Name
var studentsById = studentList.ToDictionary(keySelector: m => m.Id, elementSelector: m => m.Name);
//loop dictionary
foreach(var stu in studentsById)
{
Console.WriteLine(stu.Value);
}
}
}
public class Students{
public int Id {get;set;}
public string Name {get;set;}
}
在上面的代码中,我们首先创建“ Student”列表,其中包含一些值。
然后使用.ToDictionary()(Linq 方法)将列表 Id/Name 转换为字典、键/值对,然后循环字典以打印其值。
输出:
John
Wick
Morbius
Geralt
使用 For 循环
如果您愿意,我们可以循环每个列表项并将它们添加到字典中,而不是如上所示使用 Linq,如下所示
List<Students> studentList = new List<Students> {
new Students(){Id = 208, Name = "John"},
new Students(){Id = 209, Name = "Wick"},
new Students(){Id = 210, Name = "Morbius"},
new Students(){Id = 211, Name = "Geralt"}
};
var studentsDic = new Dictionary<int, string>();
foreach(var stu in studentList)
{
studentsDic.Add(stu.Id, stu.Name);
}
因此,如果您想查看上述代码的完整控制台应用程序示例,那就像
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
List<Students> studentList = new List<Students> {
new Students(){Id = 208, Name = "John"},
new Students(){Id = 209, Name = "Wick"},
new Students(){Id = 210, Name = "Morbius"},
new Students(){Id = 211, Name = "Geralt"}
};
var studentsDic = new Dictionary<int, string>();
foreach(var stu in studentList)
{
studentsDic.Add(stu.Id, stu.Name);
}
//loop dictionary
foreach(var stu in studentsDic)
{
Console.WriteLine(stu.Value);
}
}
}
public class Students{
public int Id {get;set;}
public string Name {get;set;}
}
输出与上面相同,因为我们正在打印字典值。
John
Wick
Morbius
Geralt
将列表转换为字典时处理重复项
将列表转换为字典时的问题之一是您可以在列表中重复值,而字典只允许添加唯一的项目,否则,它将引发如下错误
System.ArgumentException: An item with the same key has already been added.
这是图像中的示例
在上面的图像代码中,您可以看到我们重复了一个键/值 - 201/Morbius,当将其添加到字典中时,它抛出一个错误。
所以为了避免这个错误,我们可以这样做
List<Students> studentList = new List<Students> {
new Students(){Id = 208, Name = "John"},
new Students(){Id = 209, Name = "Wick"},
new Students(){Id = 210, Name = "Morbius"},
new Students(){Id = 211, Name = "Geralt"},
new Students(){Id = 210, Name = "Morbius"} // repeated value
};
var studentsDic = new Dictionary<int, string>();
var studentsById = studentList.GroupBy(m => m.Id)
.ToDictionary(keySelector: g => g.Key, elementSelector: g => g.First().Name);
//loop dictionary
foreach(var stu in studentsById)
{
Console.WriteLine(stu.Key + " "+stu.Value);
}
在上面的代码中,我们首先按“Id”对元素进行分组,然后将它们转换为字典。
另外,这里需要注意的一件事是,当我们使用时,elementSelector我们将从列表中获取第一个项目的 Name 属性。
THE END
0
二维码
海报
在 C# 中将列表转换为字典(多种方式)
在 C# 中将列表转换为字典的简单快捷方法之一是使用 Lambda
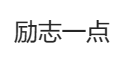