C#中的各种星形图案程序
在学习任何编程语言(如C# 、Java、C 或 C++)时,了解这些编程语言中的循环的最常见练习之一是创建星形模式程序。因此,在本文中,我提供了各种 C# 星形和金字塔图案程序。
1.使用C#创建简单的金字塔星形图案
在此示例中,我们将在 C# 中使用星号 (*) 创建简单的金字塔等边三角形图案。
using System;
public class TriangleProgram
{
public static void Main()
{
Console.WriteLine("Program for displaying pattern of *.");
Console.Write("Enter the maximum number of * line:");
int n = Convert.ToInt32(Console.ReadLine());
//for loop to work until i = n
for (int i = 1; i <= n; i++)
{
//for loop to add space
for (int j = 1; j <= (n - i); j++)
{
Console.Write(" ");
}
//for loop to print *, for each line
//k < i *2
//so for example, if i =1, it will print star for 1 times, as loop will work until k < 2, that is 1 time
// when i = 2, condition will be k < 4, means it will print star for 3 times
// and so on.
for (int k = 1; k < i * 2; k++)
{
Console.Write("*");
}
Console.WriteLine();
}
}
}
输出:
Program for displaying pattern of *.
Enter the maximum number of * line:5
*
***
*****
*******
*********
在上面的代码中,我们使用了 3 个 for 循环,其中一个是主循环,它将循环直到 i 的值不等于 n
第二个循环用于在打印星号“*”之前包含空格。
第三个循环在代码注释中进行了解释,它用于打印“*”并创建所需的模式。
2. C#中使用*打印直角三角形图案
using System;
public class RightAngleTrianglePatternProgram
{
public static void Main()
{
for (int i = 1; i <= 6; i++)
{
for (int j = 1; j <= i; j++)
{
Console.Write("*");
}
Console.WriteLine();
}
}
}
输出:
*
**
***
****
*****
******
这个例子比较容易理解,在上面的代码中,我们使用了两个for循环。
循环 1 运行直到 i 的值小于或等于 6,这意味着我们将创建 6 行来打印星号。
循环2打印星号,每当执行此循环时,它将打印第一行的1列,因为i = 1,j = 1,当i = 2的值时,它将打印2次,因为循环可以运行两次等等。
3.倒直角三角形
在此示例中,我们将创建倒直角三角形。
using System;
public class InvertedRightAngleTriangleProgram
{
public static void Main()
{
//loop to start with 8, this time as we will print inverted triangle
//loop to print number of rows, here 8
for (int row = 8; row >= 1; row--)
{
//loop to print columns
for (int col = 1; col <= row; col++)
{
Console.Write("*");
}
// to place cursor to next line
Console.WriteLine();
}
}
}
输出:
********
*******
******
*****
****
***
**
*
4. C#中使用星形的菱形图案
在此程序中,我们将代码分为两部分,第一部分将打印等边三角形,第二部分将打印倒等边三角形
using System;
public class DiamondTriangleProgram
{
public static void Main()
{
Console.WriteLine("Program for displaying pattern of *.");
Console.Write("Enter the maximum number of *: (Top to Middle line)");
int n = Convert.ToInt32(Console.ReadLine());
//loop to print first half or equilateral triangle
for (int i = 1; i <= n; i++)
{
//for loop to add space
for (int j = 1; j <= (n - i); j++)
{
Console.Write(" ");
}
//for loop to print *, for each line
//k < i *2
//so for example, if i =1, it will print star for 1 times, as loop will work until k < 2, that is 1 time
// when i = 2, condition will be k < 4, means it will print star for 3 times
// and so on.
for (int k = 1; k < i * 2; k++) { Console.Write("*"); } Console.WriteLine(); } //loop to print inverted equilateral triangle for (int i = n - 1; i >= 1; i--)
{
//for loop to add space
for (int j = 1; j <= (n - i); j++)
{
Console.Write(" ");
}
//for loop to print *, for each line
//k < i *2
//so for example, if i =1, it will print star for 1 times, as loop will work until k < 2, that is 1 time
// when i = 2, condition will be k < 4, means it will print star for 3 times
// and so on.
for (int k = 1; k < i * 2; k++)
{
Console.Write("*");
}
Console.WriteLine();
}
Console.WriteLine();
}
}
输出:
Program for displaying pattern of *.
Enter the maximum number of *: (Top to Middle line)6
*
***
*****
*******
*********
***********
*********
*******
*****
***
*
THE END
0
二维码
海报
C#中的各种星形图案程序
在学习任何编程语言(如C# 、Java、C 或 C++)时,了解这些编程语言中的循环的最常见练习之一是创建星形模式程序。因此,在本文中,我提供了各种 C# 星形和金字塔图案程序。
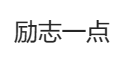