使用Datatable将html表导出为excel、pdf、csv格式
您知道如何使用 Datatable 将 html 表格导出为 excel、pdf、CSV 或 excel 格式吗?如果你不这样做,我今天就解释一下。
我们可以用数据表做很多事情。那么让我解释一下如何将 HTML 表格导出到 Excel。
什么是数据表
Datatable 是一个与 HTML 表格配合使用的 JavaScript 库。您可以使用数据表对 HTML 表进行排序、分页、搜索、导出等操作。听起来很有趣吧?在了解与您的网站集成有多么容易后,您可能会想知道。
那么我们开始吧?
所以首先你必须创建一个 HTML 表格
<table class="display nowrap" id="example" style="width:100%">
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Age</th>
<th>Start date</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Nixon</td>
<td>System Architect</td>
<td>Edinburgh</td>
<td>61</td>
<td>2011-04-25</td>
<td>$320,800</td>
</tr>
<tr>
<td>Garrett Thomas</td>
<td>Accountant</td>
<td>Tokyo</td>
<td>63</td>
<td>2011-07-25</td>
<td>$170,750</td>
</tr>
<tr>
<td>Ashton Fox</td>
<td>Junior Technical Author</td>
<td>San Francisco</td>
<td>66</td>
<td>2009-01-12</td>
<td>$86,000</td>
</tr>
</tbody>
</table>
这是我们的示例 HTML 表格。这里没有什么特别的,就像你的普通 HTML 表格一样。
现在我们必须在代码中添加一些 CSS 和 Javascript 依赖项。是的,这很重要。
您可以将这些 CSS 文件添加到表格上方
<link href="https://cdn.datatables.net/buttons/2.2.3/css/buttons.dataTables.min.css" rel="stylesheet" type="text/css">
<link href="https://cdn.datatables.net/1.12.1/css/jquery.dataTables.min.css" rel="stylesheet" type="text/css">
并将这些 Javascript 文件添加到表格下方
<script src="https://code.jquery.com/jquery-3.5.1.js"></script>
<script src="https://cdn.datatables.net/1.12.1/js/jquery.dataTables.min.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/dataTables.buttons.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.1.3/jszip.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/pdfmake.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/vfs_fonts.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/buttons.html5.min.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/buttons.print.min.js"></script>
现在在 javascript 库下面添加 javascript 代码
<script>
$(document).ready(function() {
$('#example').DataTable( {
searching: true,
info : true,
paging: true,
dom: 'Bfrtip',
buttons: [
'copy', 'csv', 'excel', 'pdf'
]
} );
} );
</script>
现在我们进入最后一部分..只需将 id 分配给表即可。
让我们仔细看看表功能控制器。
这是单个文件中的最终代码:将 HTML 表导出到 Excel
<link href="https://cdn.datatables.net/buttons/2.2.3/css/buttons.dataTables.min.css" rel="stylesheet" type="text/css">
<link href="https://cdn.datatables.net/1.12.1/css/jquery.dataTables.min.css" rel="stylesheet" type="text/css">
<table class="display nowrap" id="example" style="width:100%">
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Age</th>
<th>Start date</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Nixon</td>
<td>System Architect</td>
<td>Edinburgh</td>
<td>61</td>
<td>2011-04-25</td>
<td>$320,800</td>
</tr>
<tr>
<td>Garrett Thomas</td>
<td>Accountant</td>
<td>Tokyo</td>
<td>63</td>
<td>2011-07-25</td>
<td>$170,750</td>
</tr>
<tr>
<td>Ashton Fox</td>
<td>Junior Technical Author</td>
<td>San Francisco</td>
<td>66</td>
<td>2009-01-12</td>
<td>$86,000</td>
</tr>
</tbody>
</table>
<script src="https://code.jquery.com/jquery-3.5.1.js"></script>
<script src="https://cdn.datatables.net/1.12.1/js/jquery.dataTables.min.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/dataTables.buttons.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.1.3/jszip.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/pdfmake.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/vfs_fonts.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/buttons.html5.min.js"></script>
<script src="https://cdn.datatables.net/buttons/2.2.3/js/buttons.print.min.js"></script>
<script>
$(document).ready(function() {
$('#example').DataTable( {
searching: true,
info : true,
paging: true,
dom: 'Bfrtip',
buttons: [
'copy', 'csv', 'excel', 'pdf'
]
} );
} );
</script>
THE END
0
二维码
海报
使用Datatable将html表导出为excel、pdf、csv格式
您知道如何使用 Datatable 将 html 表格导出为 excel、pdf、CSV 或 excel 格式吗?如果你不这样做,我今天就解释一下。
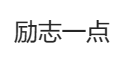