在PHP 中调整图像大小
在 PHP 中调整图像大小的一个简单方法是使用 GD 库:
$original = imagecreatefromjpeg("ORIGINAL.jpg");
$resized = imagecreatetruecolor(NEW WIDTH, NEW HEIGHT);
imagecopyresampled($resized, $original, 0, 0, 0, 0, NEW WIDTH, NEW HEIGHT, WIDTH, HEIGHT);
imagejpeg($resized, "RESIZED.jpg");
1.简单的图像调整大小
1-basic-resize.php
<?php
// (A) READ THE ORIGINAL IMAGE
$original = imagecreatefrompng("PHP-resize-a.png"); // original 600 x 400 px
// (B) EMPTY CANVAS WITH REQUIRED DIMENSIONS
// IMAGECREATETRUECOLOR(WIDTH, HEIGHT)
$resized = imagecreatetruecolor(300, 200); // smaller by 50%
// (C) RESIZE THE IMAGE
// IMAGECOPYRESAMPLED(TARGET, SOURCE, TX, TY, SX, SY, TWIDTH, THEIGHT, SWIDTH, SHEIGHT)
imagecopyresampled($resized, $original, 0, 0, 0, 0, 300, 200, 600, 400);
// (D) SAVE/OUTPUT RESIZED IMAGE
imagepng($resized, "demoA.png");
// (E) OPTIONAL - CLEAN UP
imagedestroy($original);
imagedestroy($resized);
这是上面介绍的实际工作版本,应该非常简单明了。要调整图像大小 - 我们只需创建一个空画布,然后复制源图像并调整其大小。
2.调整透明图像的大小
2-transparent-resize.php
<?php
// (A) READ THE ORIGINAL IMAGE
$original = imagecreatefrompng("PHP-resize-b.png"); // original 512 x 512 px
// (B) EMPTY CANVAS WITH REQUIRED DIMENSIONS
$resized = imagecreatetruecolor(256, 256); // smaller by 50%
// (C) PREFILL WITH TRANSPARENT LAYER
imagealphablending($resized, false);
imagesavealpha($resized, true);
imagefilledrectangle(
$resized, 0, 0, 256, 256,
imagecolorallocatealpha($resized, 255, 255, 255, 127)
);
// (D) RESIZE THE IMAGE
imagecopyresampled($resized, $original, 0, 0, 0, 0, 256, 256, 512, 512);
// (E) SAVE/OUTPUT RESIZED IMAGE
imagepng($resized, "demoB.png");
// (F) OPTIONAL - CLEAN UP
imagedestroy($original);
imagedestroy($resized);
是的,几乎一样。我们只添加了一个额外的部分(C)- 将$resized画布设置为 alpha(透明),并在调整大小之前用透明层填充它。
3.调整图像大小功能
3-resizer.php
<?php
function resizer ($source, $destination, $size, $quality=null) {
// $source - Original image file
// $destination - Resized image file name
// $size - Single number for percentage resize
// Array of 2 numbers for fixed width + height
// $quality - Optional image quality. JPG & WEBP = 0 to 100, PNG = -1 to 9
// (A) CHECKS
$allowed = ["bmp", "gif", "jpg", "jpeg", "png", "webp"];
$sExt = strtolower(pathinfo($source)["extension"]);
$dExt = strtolower(pathinfo($destination)["extension"]);
if (!in_array($sExt, $allowed)) { throw new Exception("$sExt - Invalid image file type"); }
if (!in_array($dExt, $allowed)) { throw new Exception("$dExt - Invalid image file type"); }
if (!file_exists($source)) { throw new Exception("Source image file not found"); }
// (B) IMAGE DIMENSIONS
$dimensions = getimagesize($source);
$width = $dimensions[0];
$height = $dimensions[1];
if (is_array($size)) {
$new_width = $size[0];
$new_height = $size[1];
} else {
$new_width = ceil(($size/100) * $width);
$new_height = ceil(($size/100) * $height);
}
// (C) RESIZE
// (C1) RESPECTIVE PHP IMAGE FUNCTIONS
$fnCreate = "imagecreatefrom" . ($sExt=="jpg" ? "jpeg" : $sExt);
$fnOutput = "image" . ($dExt=="jpg" ? "jpeg" : $dExt);
// (C2) IMAGE OBJECTS
$original = $fnCreate($source);
$resized = imagecreatetruecolor($new_width, $new_height);
// (C3) TRANSPARENT IMAGES ONLY
if ($sExt=="png" || $sExt=="gif") {
imagealphablending($resized, false);
imagesavealpha($resized, true);
imagefilledrectangle(
$resized, 0, 0, $new_width, $new_height,
imagecolorallocatealpha($resized, 255, 255, 255, 127)
);
}
// (C4) COPY & RESIZE
imagecopyresampled(
$resized, $original, 0, 0, 0, 0,
$new_width, $new_height, $width, $height
);
// (D) OUTPUT & CLEAN UP
if (is_numeric($quality)) {
$fnOutput($resized, $destination, $quality);
} else {
$fnOutput($resized, $destination);
}
imagedestroy($original);
imagedestroy($resized);
}
// (E) EXAMPLE USAGE
// (E1) PERCENTAGE RESIZE
resizer("PHP-resize-a.png", "demoC.jpg", 50);
resizer("PHP-resize-b.png", "demoD.png", 25);
// (E2) FIXED DIMENSION RESIZE + QUALITY
resizer("PHP-resize-a.png", "demoE.jpg", [150, 100], 60);
resizer("PHP-resize-b.png", "demoF.webp", [128, 128], 70);
最后,这个函数为你们在自己的项目中使用提供了一个小小的便利——基本上,是上述示例的“智能版本”,可以进行检查和自动尺寸计算。虽然不是世界上最好的,但应该足以启动。
THE END
0
二维码
海报
在PHP 中调整图像大小
在 PHP 中调整图像大小的一个简单方法是使用 GD 库
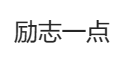