如何在 PHP 中从数据库获取和更新数据?
本教程重点介绍如何使用 PHP 从数据库中获取和更新数据。您将学习如何使用 PHP 从数据库获取和更新数据。我们将使用如何在 PHP 中从 MySQL 数据库获取和更新数据。如果您对如何使用 PHP 从 MySQL 数据库获取和更新数据有疑问,那么我将给出一个简单的示例和解决方案。
现在,让我们看看如何使用 PHP 从 MySQL 数据库获取和更新数据的文章。这是一个简单的示例,说明如何使用 PHP 从 MySQL 数据库获取和更新数据。您可以了解如何使用 PHP 从数据库获取和更新数据的概念。如果您对如何在 PHP 中从数据库获取和更新数据有疑问,那么我将给出一个简单的示例和解决方案。
第1步:创建数据库连接文件
<?php
php
$servername='localhost';
='localhost';
$username='root';
='root';
$password='';
='';
$dbname = "my_db";
= "my_db";
$conn=mysqli_connect($servername,$username,$password,$dbname);
=mysqli_connect($servername,$username,$password,$dbname);
if(!$conn){
if(!$conn){
die('Could not Connect MySql Server:' .mysql_error());
die('Could not Connect MySql Server:' .mysql_error());
}
}
?>
步骤2:从数据库中获取数据
<!DOCTYPE html>
<html lang="en">
lang="en">
<head>
<meta charset="utf-8">
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>PHP code to retrieve and update data from MySQL database</title>
<title>PHP code to retrieve and update data from MySQL database</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round|Open+Sans">
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round|Open+Sans">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
<style type="text/css">
<style type="text/css">
.bs-example{
.bs-example{
margin: 20px;
: 20px;
}
}
</style>
</style>
<script type="text/javascript">
<script type="text/javascript">
$(document).ready(function(){
(document).ready(function(){
$('[data-toggle="tooltip"]').tooltip();
('[data-toggle="tooltip"]').tooltip();
});
});
</script>
</script>
</head>
<body>
<div class="bs-example">
<div class="bs-example">
<div class="container">
<div class="container">
<div class="row">
<div class="row">
<div class="col-md-12">
<div class="col-md-12">
<div class="page-header clearfix">
<div class="page-header clearfix">
<h2 class="pull-left">Users List</h2>
<h2 class="pull-left">Users List</h2>
</div>
</div>
<?php
<?php
include_once 'db.php';
'db.php';
$result = mysqli_query($conn,"SELECT * FROM users");
= mysqli_query($conn,"SELECT * FROM users");
?>
?>
<?php
<?php
if (mysqli_num_rows($result) > 0) {
if (mysqli_num_rows($result) > 0) {
?>
?>
<table class='table table-bordered table-striped'>
<table class='table table-bordered table-striped'>
<tr>
<tr>
<td>Name</td>
<td>Name</td>
<td>Email id</td>
<td>Email id</td>
<td>Mobile</td>
<td>Mobile</td>
<td>Action</td>
<td>Action</td>
</tr>
</tr>
<?php
<?php
$i=0;
=0;
while($row = mysqli_fetch_array($result)) {
while($row = mysqli_fetch_array($result)) {
?>
?>
<tr>
<tr>
<td><?php echo $row["name"]; ?></td>
<td><?php echo $row["name"]; ?></td>
<td><?php echo $row["email"]; ?></td>
<td><?php echo $row["email"]; ?></td>
<td><?php echo $row["mobile"]; ?></td>
<td><?php echo $row["mobile"]; ?></td>
<td><a href="update-action.php?userid=<?php echo $row["id"]; ?>">Update</a></td>
<td><a href="update-action.php?userid=<?php echo $row["id"]; ?>">Update</a></td>
</tr>
</tr>
<?php
<?php
$i++;
++;
}
}
?>
?>
</table>
</table>
<?php
<?php
}else{
}else{
echo "No result found";
"No result found";
}
}
?>
?>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
步骤 3:从数据库更新数据
<?php
php
// Include database connection file
// Include database connection file
require_once "connection.php";
"connection.php";
if(count($_POST)>0) {
if(count($_POST)>0) {
mysqli_query($conn,"UPDATE users set name='" . $_POST['name'] . "', mobile='" . $_POST['mobile'] . "' ,email='" . $_POST['email'] . "' WHERE id='" . $_POST['id'] . "'");
($conn,"UPDATE users set name='" . $_POST['name'] . "', mobile='" . $_POST['mobile'] . "' ,email='" . $_POST['email'] . "' WHERE id='" . $_POST['id'] . "'");
$message = "Record Modified Successfully";
= "Record Modified Successfully";
}
}
$result = mysqli_query($conn,"SELECT * FROM users WHERE id='" . $_GET['userid'] . "'");
= mysqli_query($conn,"SELECT * FROM users WHERE id='" . $_GET['userid'] . "'");
$row= mysqli_fetch_array($result);
= mysqli_fetch_array($result);
?>
<!DOCTYPE html>
<html lang="en">
lang="en">
<head>
<meta charset="UTF-8">
<meta charset="UTF-8">
<title>Update Record</title>
<title>Update Record</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.css">
<style type="text/css">
<style type="text/css">
.wrapper{
.wrapper{
width: 500px;
: 500px;
margin: 0 auto;
: 0 auto;
}
}
</style>
</style>
</head>
<body>
<div class="wrapper">
<div class="wrapper">
<div class="container-fluid">
<div class="container-fluid">
<div class="row">
<div class="row">
<div class="col-md-12">
<div class="col-md-12">
<div class="page-header">
<div class="page-header">
<h2>Update Record</h2>
<h2>Update Record</h2>
</div>
</div>
<p>Please edit the input values and submit them to update the record.</p>
<p>Please edit the input values and submit them to update the record.</p>
<form action="<?php echo htmlspecialchars(basename($_SERVER['REQUEST_URI'])); ?>" method="post">
<?php echo htmlspecialchars(basename($_SERVER['REQUEST_URI'])); ?>" method="post">
<div class="form-group">
<div class="form-group">
<label>Name</label>
<label>Name</label>
<input type="text" name="name" class="form-control" value="<?php echo $row["name"]; ?>">
<?php echo $row["name"]; ?>">
</div>
</div>
<div class="form-group ">
<div class="form-group ">
<label>Email</label>
<label>Email</label>
<input type="email" name="email" class="form-control" value="<?php echo $row["email"]; ?>">
<?php echo $row["email"]; ?>">
</div>
</div>
<div class="form-group">
<div class="form-group">
<label>Mobile</label>
<label>Mobile</label>
<input type="mobile" name="mobile" class="form-control" value="<?php echo $row["mobile"]; ?>">
<?php echo $row["mobile"]; ?>">
</div>
</div>
<input type="hidden" name="id" value="<?php echo $row["id"]; ?>"/>
<?php echo $row["id"]; ?>"/>
<input type="submit" class="btn btn-primary" value="Submit">
<input type="submit" class="btn btn-primary" value="Submit">
<a href="users.php" class="btn btn-default">Cancel</a>
<a href="users.php" class="btn btn-default">Cancel</a>
</form>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
THE END
0
二维码
海报
如何在 PHP 中从数据库获取和更新数据?
本教程重点介绍如何使用 PHP 从数据库中获取和更新数据。您将学习如何使用 PHP 从数据库获取和更新数据。
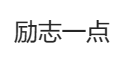