使用 PHP 从 MySQL 数据库存储和检索图像
一般来说,当我们在PHP中上传图片文件时,上传的图片会存储在服务器的某个目录中,并且相应的图片名称会存储在数据库中。显示时,从服务器检索文件并将图像呈现在网页上。但是,如果不想消耗服务器空间,文件可以只存储在数据库中。您可以上传图像,而无需使用 MySQL 数据库将文件物理存储在服务器上。使用 PHP 和 MySQL 在数据库中存储和检索图像非常容易。
如果您担心服务器空间并且需要服务器上的可用空间,您可以将图像文件直接插入数据库中,而无需将其上传到服务器的目录中。此过程有助于优化服务器空间,因为图像文件内容存储在数据库中而不是服务器中。在本教程中,我们将向您展示如何将图像文件存储到 MySQL 数据库中并使用 PHP 从数据库中检索图像。
在开始将文件上传与数据库集成之前,先看一下文件结构。
store_retrieve_image_from_database/
├── dbConfig.php
├── index.php
├── upload.php
├── view.php
└── css/
└── style.css
在 MySQL 中插入图像文件
MySQL有BLOB(二进制大对象)数据类型,可以保存大量的二进制数据。BLOB 数据类型非常适合在数据库中存储图像数据。在 MySQL 中,有四种 BLOB 类型可用:TINYBLOB、BLOB、MEDIUMBLOB 和 LONGBLOB。LONGBLOB 数据类型非常适合存储图像文件数据。
创建数据库表
为了存储文件内容,数据库中需要有一个表。以下 SQL在 MySQL 数据库中创建一个images具有LONGBLOB 数据类型字段的表。
CREATE TABLE `images` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`image` longblob NOT NULL,
`created` datetime NOT NULL DEFAULT current_timestamp(),
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
数据库配置(dbConfig.php)
该dbConfig.php文件用于连接和选择数据库。根据您的 MySQL 数据库凭据指定数据库主机 ( $dbHost)、用户名 ( $dbUsername)、密码 ( $dbPassword) 和名称 ( )。$dbName
<?php
// Database configuration
$dbHost = "localhost";
$dbUsername = "root";
$dbPassword = "root";
$dbName = "codexworld_db";
// Create database connection
$db = new mysqli($dbHost, $dbUsername, $dbPassword, $dbName);
// Check connection
if ($db->connect_error) {
die("Connection failed: " . $db->connect_error);
}
图片上传表格
创建一个带有文件输入字段的 HTML 表单,用于选择要上传的图像文件。确保<form>标签包含以下属性。
method="post"
enctype="multipart/form-data"
<form action="upload.php" method="post" enctype="multipart/form-data">
<label>Select Image File:</label>
<input type="file" name="image">
<input type="submit" name="submit" value="Upload">
</form>
将图像文件存储在数据库中(upload.php)
该upload.php文件处理图像上传和数据库插入过程。
检查用户是否选择上传图片文件。
使用PHP file_get_contents()函数通过 tmp_name 检索图像文件的内容。
使用 PHP 和 MySQL 将图像的二进制内容插入数据库。
向用户显示图像上传状态。
<?php
// Include the database configuration file
require_once 'dbConfig.php';
// If file upload form is submitted
$status = $statusMsg = '';
if(isset($_POST["submit"])){
$status = 'error';
if(!empty($_FILES["image"]["name"])) {
// Get file info
$fileName = basename($_FILES["image"]["name"]);
$fileType = pathinfo($fileName, PATHINFO_EXTENSION);
// Allow certain file formats
$allowTypes = array('jpg','png','jpeg','gif');
if(in_array($fileType, $allowTypes)){
$image = $_FILES['image']['tmp_name'];
$imgContent = addslashes(file_get_contents($image));
// Insert image content into database
$insert = $db->query("INSERT into images (image, created) VALUES ('$imgContent', NOW())");
if($insert){
$status = 'success';
$statusMsg = "File uploaded successfully.";
}else{
$statusMsg = "File upload failed, please try again.";
}
}else{
$statusMsg = 'Sorry, only JPG, JPEG, PNG, & GIF files are allowed to upload.';
}
}else{
$statusMsg = 'Please select an image file to upload.';
}
}
// Display status message
echo $statusMsg;
?>
从数据库检索图像(view.php)
在该view.php文件中,我们将从 MySQL 数据库中检索图像内容并将其列出在网页上。
src 属性中的 data、charset 和 base64 参数用于显示来自 MySQL 数据库的图像 BLOB。
<?php
// Include the database configuration file
require_once 'dbConfig.php';
// Get image data from database
$result = $db->query("SELECT image FROM images ORDER BY id DESC");
?>
<!-- Display images with BLOB data from database -->
<?php if($result->num_rows > 0){ ?>
<div class="gallery">
<?php while($row = $result->fetch_assoc()){ ?>
<img src="data:image/jpg;charset=utf8;base64,<?php echo base64_encode($row['image']); ?>" />
<?php } ?>
</div>
<?php }else{ ?>
<p class="status error">Image(s) not found...</p>
<?php } ?>
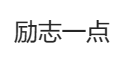